Quickstart
Minimal Animation Script
A minimal animation script looks something like this:
1import ananimlib as al
2
3al.Animate(
4 al.AddAnObject(al.Rectangle([1,1])),
5 al.Wait(1.0)
6)
7
8al.play_movie()
The output is a one second “animation” of the outline of a white rectangle sitting motionless in the center of the canvas.

Let’s take a look at the code.
In Line 1, we import ananimlib under the alias ‘al’ to save us some keystrokes and because “Namespaces are one honking great idea.”
In line 3, we invoke the library’s Animate method to start rendering frames. Animate takes a variable number of Instructions and executes them sequentially to create the final animation.
On line 8, we create an AddAnObject instruction to Add an Animation Object to the scene.
AddAnObject takes an AnObject (Animation Object) as its input. In this case, we create a 1x1 Rectangle.
AnObjects added to the scene will appear in each frame of the animation unless they go off camera or are removed with RemoveAnObject.
Line 5 issues the Wait instruction which simply waits for the specified amount of time without making any changes to the scene. Without the wait instruction in this example, our animation would not contain any output frames since AddAnObject takes zero seconds of animation time.
Finally, Line 8 plays the resulting animation.
An Actual Animation
Our first example wasn’t much of an animation given that the rectangle didn’t actually move. Let’s make it move across the canvas.
1import ananimlib as al
2
3al.Animate(
4 al.AddAnObject(al.Rectangle([1,1]), key = "rect"),
5 al.MoveTo("rect", [-3,0]),
6 al.Move("rect", [6,0], duration=1.0),
7 al.Wait(1.0),
8)
9
10al.play_movie()
At line 4, we’ve added the optional input key to AddAnObject which allows us to refer to the rectangle by the string “rect” in later instructions.
At Line 5, we inserted a MoveTo instruction. This moves our rectangle to absolute coordinates [-3,0]. MoveTo takes an optional duration argument that dictates how much animation time the move will take. The default is 0.0 seconds so in this case, the move is instantaneous.
At Line 6, we inserted a Move instruction, which represents a move relative to the AnObject’s current location. This moves our rectangle 6 units horizontally and zero units vertically. Here we’ve set the optional duration parameter to 1.0 so the move occurs over 1.0 second of animation time.
Here’s the output

Simultaneous Instructions
What if we want the rectangle to spin 360 degrees at the same time that it’s moving across the canvas? The RunParallel instruction allows you to execute a set of instructions simultaneoulsly.
1import ananimlib as al
2
3rect = al.Rectangle([1,1])
4
5al.Animate(
6 al.AddAnObject(rect),
7 al.MoveTo(rect,[-3.0,0.0]),
8 al.RunParallel(
9 al.Move(rect, [6,0], duration=1.0),
10 al.Rotate(rect, 2*3.1415, duration=1.0),
11 ),
12 al.Wait(1.0)
13)
14
15al.play_movie()
In this example, we instantiated our rectangle on Line 3 and passed a reference to AddAnObject on Line 6. We also passed a reference to the instructions at line 7, 9, and 10 rather than the String based key that we used in the previous example. You can refer to AnObjects in the scene either by reference or by key, if one was assigned.
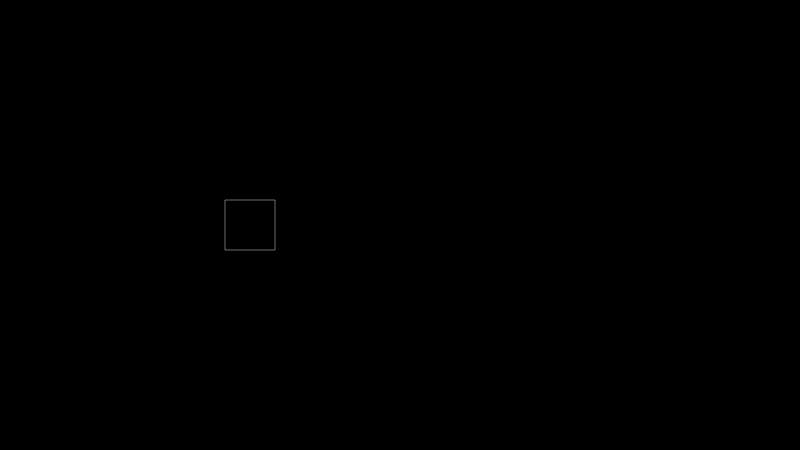
The RunParallel instruction can be combined with the RunSequential instruction to achieve a variety of effects, like making two sequential moves while simultaneously executing a full rotation.
1import ananimlib as al
2
3rect = al.Rectangle([1,1])
4
5al.Animate(
6 al.AddAnObject(al.Rectangle([1,1]), "rect"),
7 al.MoveTo("rect", [-3.0,0.0]),
8 al.RunParallel(
9 al.RunSequential(
10 al.Move("rect", [3, 2], duration=0.5),
11 al.Move("rect", [3,-2], duration=0.5),
12 ),
13 al.Rotate("rect", 2*3.1415, duration=1.0),
14 ),
15 al.Wait(1.0)
16)
17
18al.play_movie()

You can also use RunSequential and RunParallel to build complex instructions from simpler instructions.
1import ananimlib as al
2
3
4def double_move(key, pos1, pos2, duration):
5 return al.RunSequential(
6 al.Move(key, pos1, duration=duration/2),
7 al.Move(key, pos2, duration=duration/2)
8 )
9
10al.Animate(
11 al.AddAnObject(al.Rectangle([1,1]), "rect"),
12 al.MoveTo("rect", [-3.0,0.0]),
13 al.RunParallel(
14 double_move("rect",[3,2],[3,-2],duration=1.0),
15 al.Rotate("rect", 2*3.1415, duration=1.0),
16 ),
17 al.Wait(1.0),
18 double_move("rect",[-3,2],[-3,-2],duration=1.0),
19 al.Wait(1.0)
20)
21
22al.play_movie()

The About Point
Consider the following script. Lines 8 and 9 rotate the rectangle 180 degrees (pi radians) counter-clockwise and then back to zero. Lines 14 and 15 do exactly the same, but the rectangle sweeps out an arc rather than rotating about its center. This behavior is the result of the SetAboutPoint instruction at line 12. All position, rotation, and scale operations are performed with respect to an AnObject’s about point.
1import ananimlib as al
2
3rect = al.Rectangle([1,1])
4
5al.Animate(
6 al.AddAnObject(rect),
7
8 al.Rotate(rect, 3.14, duration=1.0),
9 al.Rotate(rect, 0, duration=1.0),
10 al.Wait(1.0),
11
12 al.SetAboutPoint(rect, [-2,-1]),
13
14 al.Rotate(rect, 3.14, duration=1.0),
15 al.Rotate(rect, 0, duration=1.0),
16
17 al.Wait(1.0)
18)
19
20al.play_movie()

Below, we display the underlying coordinate grid as well as the rectangle’s about point to make it easier to see how the rotation relates to the about point.

The about point is defined with respect to the AnObject’s internal coordinate system. In the next example, the move on line 9 is with respect to the center of the rectangle. On line 12, the rectangle’s about point is moved to [-2,-1] with respect to its own coordinate system so that the move to [0,0] on line 14 puts the center of the rectangle at [2,1] with respect to the main coordinate grid.
1import ananimlib as al
2
3a = al.AnEngine()
4
5rect = al.Rectangle([1,1])
6
7al.Animate(
8 al.AddAnObject(rect),
9 al.MoveTo(rect,[3,2],duration=1.0),
10 al.Wait(1.0),
11
12 al.SetAboutPoint(rect, [-2,-1]),
13
14 al.MoveTo(rect,[0,0],duration=1.0),
15 al.Wait(1.0)
16)
17
18al.play_movie()

Here’s the output again with the coordinate grid and the about point displayed.
The Composite AnObject
CompositAnObject contains a collection of AnObjects which can then be manipulated separately or as a group. Here’s an example:
1import ananimlib as al
2
3composite = al.CompositeAnObject()
4composite.add_anobject(al.Rectangle([1,1]), 'rect')
5composite.add_anobject(al.Arrow([0,0],[2,0]), 'arrow')
6
7al.Animate(
8 al.AddAnObject(composite),
9 al.MoveTo(composite,[3,0], duration=1.0),
10 al.Wait(0.5),
11
12 al.MoveTo([composite,'arrow'], [0.5,0],duration=1.0),
13 al.Wait(0.5),
14
15 al.SetAboutPoint([composite,'arrow'], [-0.5,0.0]),
16 al.Rotate([composite,'arrow'], 3.1415,duration=1.0),
17 al.Wait(0.5),
18
19 al.MoveTo(composite,[0,0],duration=1.0),
20 al.Wait(0.5),
21)
22
23al.play_movie()
In lines 3,4, and 5, we construct a CompositeAnObject to which we add a Rectangle and an Arrow.
The MoveTo at line 9 moves the entire composite to position [3,0] with respect to the main canvas.
The MoveTo in line 12 moves just the arrow to position [0.5,0] with respect to the CompositeAnObject.
On line 15, we move the about point of the arrow to [-0.5,0.0] with respect to itself. This puts the arrow’s about point at the [0,0] with respect to the CompositeAnObject, or [3,0] with respect to the main canvas.
On line 16, we rotate the Arrow 180 degrees (or pi radians) around its about point.
Finally, the MoveTo on line 19 moves the entire composite back to the origin of the main canvas.
